How to configure JSF in Tomcat example
Introduction
Since Tomcat is not a fully fledged Java EE container we must do some additional configuration steps in order to run JSF applications. In this tutorial we will see how to do it.
This tutorial considers the following environment:
- Ubuntu 12.04
- JDK 1.7.0.21
- JSF 2.2.4
- Tomcat 7.0.35
Required Maven dependencies
Following next are the required Maven dependencies:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.byteslounge.jsf.war</groupId> <artifactId>com-byteslounge-jsf-war</artifactId> <version>1.0-SNAPSHOT</version> <packaging>war</packaging> <name>com-byteslounge-jsf-war</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>org.glassfish</groupId> <artifactId>javax.faces</artifactId> <version>2.2.4</version> </dependency> </dependencies> </project>
Note that we are including JSF dependency with compile scope (the default scope when dependency's scope is omitted) so it will be included in the application self classpath, ie. it will be bundled with the application. This is needed because Tomcat does not include the JSF runtime by default.
Configuring web.xml
The web.xml configuration we will use is very simple and just configures the Faces Servlet.
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>myapp</display-name> <servlet> <servlet-name>faces</servlet-name> <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>faces</servlet-name> <url-pattern>*.xhtml</url-pattern> </servlet-mapping> </web-app>
We are just configuring the Faces Servlet and mapping all requests which URL ends with .xhtml to be handled by the servlet.
A simple JSF managed bean
Now we define a simple JSF managed bean:
package com.byteslounge.jsf.war; import javax.annotation.PostConstruct; import javax.faces.bean.ManagedBean; import javax.faces.bean.RequestScoped; @ManagedBean @RequestScoped public class TestBean { private String message; @PostConstruct private void init() { message = "JSF is running"; } public String getMessage() { return message; } }
The managed bean has a property - message - that will be shown in the JSF view.
The JSF view
Finally we define a JSF view that will show the message returned by the managed bean:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://java.sun.com/jsf/core" xmlns:h="http://java.sun.com/jsf/html"> <h:head> <title>JSF running in Tomcat</title> </h:head> <h:body> <h:outputText value="#{testBean.message}" /> </h:body> </html>
Deploying and testing
Now we put the generated WAR file inside Tomcat's webapps folder. Remember that by default the WAR file name (excluding the .war extension) will be used as the application's context path. In this example we deployed our WAR file as myapp.war
When we access the following URL:
http://localhost:8080/myapp/pages/home.xhtml
The expected output will be generated by JSF:
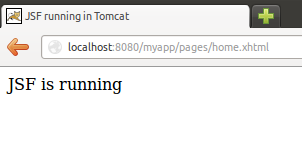