JSF dataTable example
Introduction
The dataTable component may be used to represent tabular data inside a JSF view. In this tutorial we will use a dataTable in order to present an illustrative list of users.
The model
We will need a simple model class to represent a user:
package com.byteslounge.jsf; public class User { private final String firstName; private final String lastName; private final String email; private final int age; public User(String firstName, String lastName, String email, int age) { this.firstName = firstName; this.lastName = lastName; this.email = email; this.age = age; } public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public String getEmail() { return email; } public int getAge() { return age; } }
The managed bean
We will also naturally need a managed bean:
package com.byteslounge.jsf; import java.util.ArrayList; import java.util.List; import javax.annotation.PostConstruct; import javax.faces.bean.ManagedBean; import javax.faces.bean.RequestScoped; @ManagedBean @RequestScoped public class UsersBean { private List<User> userList; @PostConstruct private void init() { userList = new ArrayList<>(); userList.add(new User("John", "Doe", "[email protected]", 32)); userList.add(new User("Peter", "Smith", "[email protected]", 25)); userList.add(new User("Mary", "Jane", "[email protected]", 27)); userList.add(new User("Mike", "Skeet", "[email protected]", 35)); } public List<User> getUserList() { return userList; } public int getUserCount() { return userList.size(); } }
We are just creating a dummy user list during bean initialization.
The dataTable
Finally the dataTable itself:
<h:dataTable value="#{usersBean.userList}" var="user" border="1"> <f:facet name="header"> <h:outputText value="Users" /> </f:facet> <h:column> <f:facet name="header"> <h:outputText value="First name" /> </f:facet> <h:outputText value="#{user.firstName}" /> </h:column> <h:column> <f:facet name="header"> <h:outputText value="Last name" /> </f:facet> <h:outputText value="#{user.lastName}" /> </h:column> <h:column> <f:facet name="header"> <h:outputText value="Email" /> </f:facet> <h:outputText value="#{user.email}" /> </h:column> <h:column> <f:facet name="header"> <h:outputText value="Age" /> </f:facet> <h:outputText value="#{user.age}" /> </h:column> <f:facet name="footer"> <h:outputText value="User count: " /> <h:outputText value="#{usersBean.userCount}" /> </f:facet> </h:dataTable>
Note the dataTable value and var properties:
value should point to a method (usually a simple getter) in our backing bean that returns the list that will be used to populate the dataTable.
var will represent each list item during the list iteration and consequently each record during table rendering.
We map each dataTable column to the corresponding user property.
The defined columns and the dataTable itself may contain facets (facets are optional). We used the header facet both for the table and the individual columns. This way we may have a global header for the whole table and individual headers for the columns.
We used a footer facet for the dataTable in order to display the user count. If we need we may also use footer facets for individual columns (ex: to display column sums) but this was not the case.
The dataTable we just defined will result in the following table being rendered:
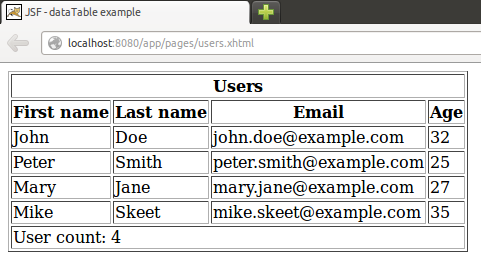