Configuring CDI with Tomcat example
Introduction
Tomcat is not a full-blown EE container so we must include some required libraries in order to enable CDI support. In this tutorial we will cover CDI configuration in Tomcat and also provide a working sample.
This tutorial considers the following software and environment:
- Ubuntu 12.04
- JDK 1.7.0.09
- Weld 1.1.10
- Tomcat 7.0.35
Configuration
Tomcat is not a full-blown EE container so we must include some required libraries in order to enable CDI support. In this tutorial we will be using Weld - the CDI reference implementation. The required Maven dependencies used in this tutorial are the following:
<dependencies> <dependency> <groupId>org.jboss.weld.servlet</groupId> <artifactId>weld-servlet</artifactId> <version>1.1.10.Final</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.0.1</version> <scope>provided</scope> </dependency> </dependencies>
In order to enable CDI we must also place an empty beans.xml file in WEB-INF folder:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/beans_1_0.xsd"> </beans>
The last configuration step is to configure our CDI listener. We do this in web.xml:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>Tomcat CDI example</display-name> <listener> <listener-class>org.jboss.weld.environment.servlet.Listener</listener-class> </listener> </web-app>
The injected bean
Now let's define the bean that will be injected by CDI in our test servlet. Following is the bean interface:
package com.byteslounge.cdi.bean; public interface Service { int doWork(int a, int b); }
Now the bean implementation:
package com.byteslounge.cdi.bean; public class ServiceBean implements Service { @Override public int doWork(int a, int b) { return a + b; } }
A simple test servlet
Now let's define a simple servlet where we will inject our bean:
package com.byteslounge.cdi.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.inject.Inject; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.byteslounge.cdi.bean.Service; @WebServlet(name = "testServlet", urlPatterns = {"/testcdi"}) public class TestServlet extends HttpServlet { private static final long serialVersionUID = 2638127270022516617L; @Inject private Service service; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { int a = 2; int b = 3; PrintWriter out = response.getWriter(); out.println("Hello World: " + service.doWork(a, b)); out.close(); } }
Note that the private property service is annotated with @Inject. This means that when the container is initializing the servlet it will look for a Service implementation. It will find our implementation - ServiceBean - and inject it into the servlet.
When we deploy the application in Tomcat and access the servlet mapped URL we will get the following output:
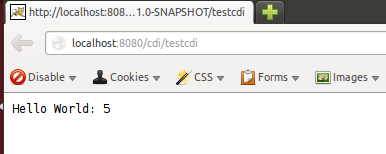
The tutorial source code is available for download at the end of this page.