How to add dependencies to a Maven project
Used software
This tutorial considers the following software and environment:
- Ubuntu 12.04
- Maven 3.0.4
- Eclipse Juno (for additional tutorial steps)
Editing your pom.xml file
In this tutorial we will be adding Apache Commons Email library as a dependency to our Maven project. Start by opening your pom.xml file and add the Commons email dependency to the project. The groupId, artifactId and version to be used in the dependency element can be easily found in the online Maven global repository or in the official webpage of the dependency provider, ie Apache dependencies info is usually available at the Apache website, Spring dependencies info is usually available at the Spring website, etc.
After editing your pom.xml and adding the Commons Email dependency, the xml file should look like the following:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <!-- ommiting other sections for clarity --> <dependencies> <!-- Commons Email dependency--> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-email</artifactId> <version>1.2</version> </dependency> </dependencies> </project>
Using the Commons Email dependency
Now that we added the Commons Email dependency to our project we can start using it in our classes:
package com.byteslounge; import org.apache.commons.mail.EmailException; import org.apache.commons.mail.SimpleEmail; public class DependencyExample { public void sendEmail() throws EmailException { SimpleEmail email = new SimpleEmail(); email.setHostName("smtphost.example.com"); email.addTo("[email protected]"); email.setFrom("[email protected]", "John Doe"); email.setSubject("Some random message"); email.setMsg("This is a random text message"); email.send(); } }
After defining the class you can issue the Maven build command. Note that the Commons Email library will be downloaded and added as a dependency to your project during the build process. Issue the following command in your project root directory:
Updating the dependency references in Eclipse
If you are using Eclipse as your IDE you must refresh the project classpath so it knows about the dependencies you just added. To acomplish this you just need to issue the following Maven command after the build process finishes:
Now the Commons Email jars are included in your project classpath:
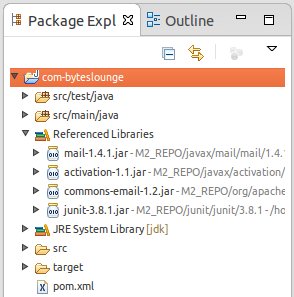